עץ בינארי
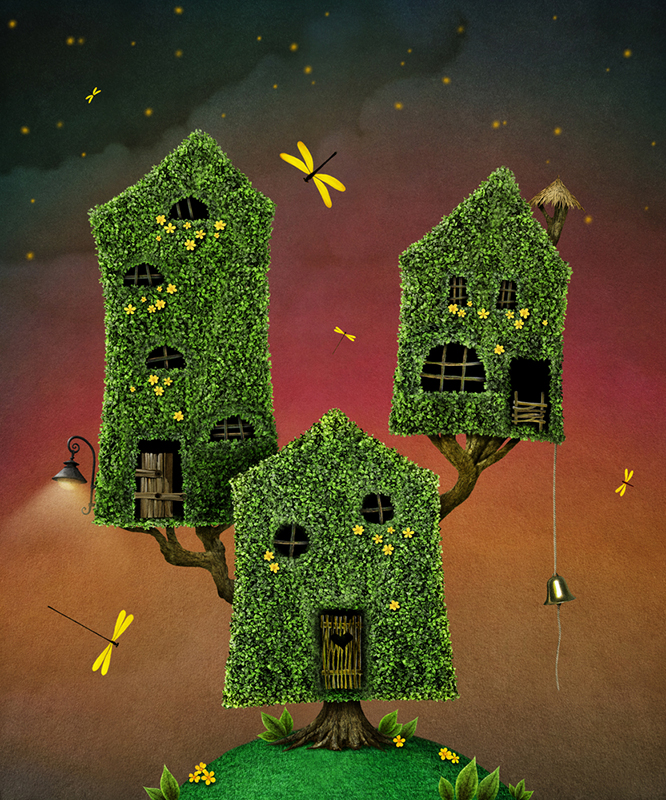
איך לבנות עץ בינארי ב - C#
- public class BinaryTreeNode {
- public int Number {get;set;};
- public BinaryTreeNode Right {get;set};
- public BinaryTreeNode Left {get;set};
- public BinaryTreeNode(int value)
- {
- Number = value;
- Right = null;
- Left = null;
- }
- }
- public class Tree {
- public BinaryTreeNode Root;
- public void InsertIntoBST(int data)
- {
- BinaryTreeNode _newNode = new BinaryTreeNode(data);
- BinaryTreeNode _current = Root;
- BinaryTreeNode _previous = _current;
- while (_current != null)
- {
- if (data < _current.Data)
- {
- _previous = _current;
- _current = _current.Left;
- }
- else if (data > _current.Data)
- {
- _previous = _current;
- _current = _current.Right;
- }
- }
- if (data < _previous.Data)
- _previous.Left = _newNode;
- else
- _previous.Right = _newNode;
- }
- }