מחשבון בצורה של מכונת סטאק
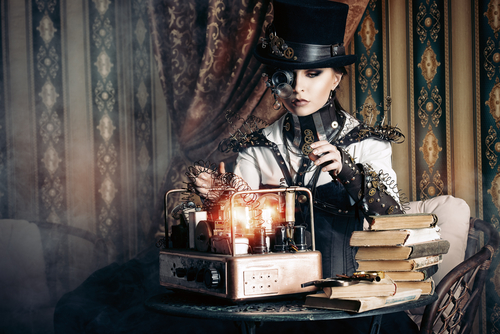
יש לכתוב קוד לחישוב נוסחה, שרשומה בכתיב פולני בעזרת שימוש בסטאק.
- static void Main(string[] args)
- {
- Console.Write(solution("512+4*+3-"));
- Console.ReadKey();
- }
- public static int solution(string S)
- {
- Stack<int> stackCreated = new Stack<int>();
- foreach (var t in S) {
- int num;
- if (int.TryParse(t.ToString(), out num))
- stackCreated.Push(num);
- else {
- int store1 = stackCreated.Pop();
- int store2 = stackCreated.Pop();
- switch (t)
- {
- case '+': store2 += store1; break;
- case '-': store2 -= store1; break;
- case '*': store2 *= store1; break;
- case '/': store2 /= store1; break;
- case '%': store2 %= store1; break;
- case '^': store2 = (int)Math.Pow(store1, store2); break;
- default: throw new Exception();
- }
- stackCreated.Push(store2);
- }
- }
- if (stackCreated.Count != 1)
- throw new Exception("Wrong input string");
- return stackCreated.Pop();
- }