מפענח מחרוזת
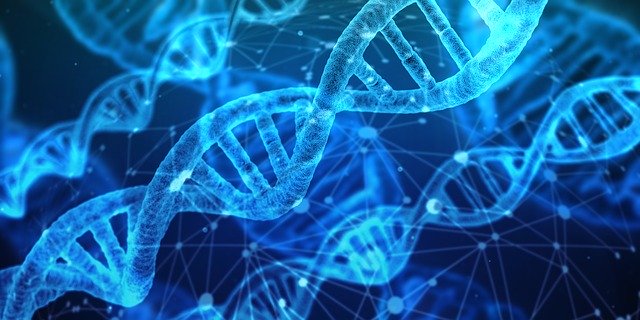
כלל הקידוד הוא: k[encoded_string] לדוגמא: s = "3[a2[c]]", return "accaccacc";
- public string DecodeString(string s) {
- string result = String.Empty;
- Stack<string> strStack = new Stack<string>();
- Stack<int> countStack = new Stack<int>();
- int i = 0;
- while(i < s.Length){
- if(char.IsDigit(s[i])) {
- int number = s[i++] - '0';
- while(char.IsDigit(s[i])) {
- number = 10 * number + s[i++] - '0';
- }
- countStack.Push(number);
- }
- else if(s[i] == '[') {
- strStack.Push(result);
- result = String.Empty;
- i++;
- }
- else if(s[i] == ']') {
- StringBuilder sb = new StringBuilder();
- int numOfTimes = countStack.Pop();
- for(int y=0; y<numOfTimes;y++) {
- sb.Append(result);
- }
- result = strStack.Pop() + sb.ToString();
- i++;
- }
- else {
- result+=s[i++];
- }
- }
- return result;
- }