deadlock
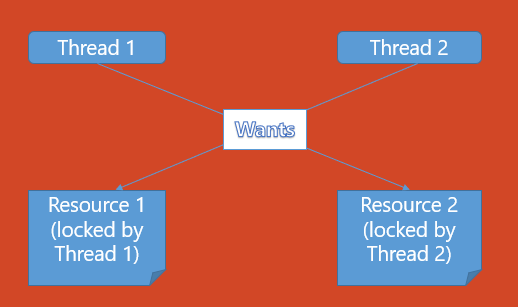
יש לכתוב דוגמה של קוד ב c#
- public class AccountManager {
- Account _fromAccount;
- Account _toAccount;
- double _amountToTransfer;
- public AccountManager(Account fromAccount, Account toAccount, double amountToTransfer) {
- this._fromAccount = fromAccount;
- this._toAccount = toAccount;
- this._amountToTransfer = amountToTransfer;
- }
- public void Transfer() {
- lock(_fromAccount) {
- Thread.Sleep(1000);
- lock(_toAccount) {
- _fromAccount.Withdraw(_amountToTransfer);
- _toAccount.Deposit(_amountToTransfer);
- }
- }
- }
- }
- public static void Main() {
- Account a = new Account(101, 5000);
- Account b = new Account(102, 3000);
- AccountManager mA = new AccountManager(a, b, 1000);
- Thread T1 = new Thread(mA.Transfer);
- AccountManager mB = new AccountManager(b, a, 2000);
- Thread T2 = new Thread(mB.Transfer);
- T1.Start();
- T2.Start();
- T1.Join();
- T2.Join();
- }
also great article can be found here: https://blog.stephencleary.com/2012/07/dont-block-on-async-code.html