איקס עיגול - לכתוב קוד שאף פעם לא הפסיד
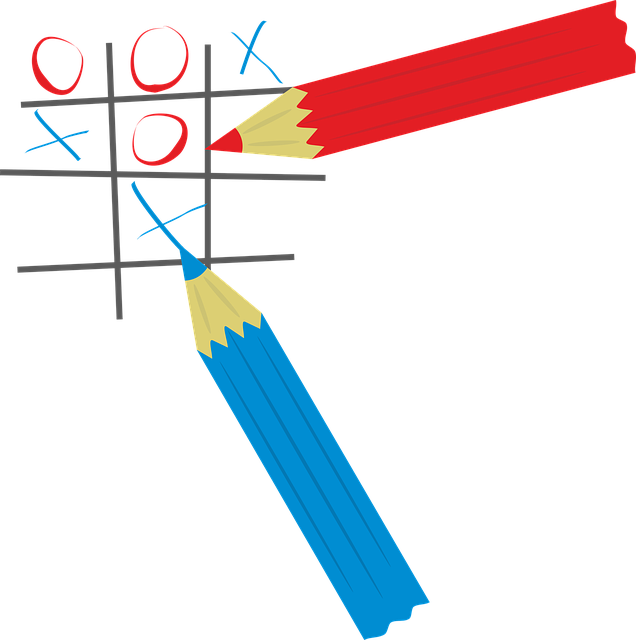
יש לכתוב פונקציה שמקבלת לוח עם מצב נתון ומס' שחקן (1 או 2) ותמאלה מקום פנוי ככה שלא תפסיד אף פעם.
- public int[] TheMoveCalculation(int[][] board, int player) {
- for (int i = 0; i < 3; i++) {
- for (int j = 0; j < 3; j++) {
- if (board[i][j] == 0) {
- board[i][j] = player;
- if (move(board, player) >= 0)
- return new[] { i, j };
- board[i][j] = 0;
- }
- }
- }
- return null; // never will be here, because this is always option for win or remis in this game
- }
- private int move(int[][] board, int player) { // 1-win, 0-remis, -1-loose
- int free = 0;
- for (int i = 0; i < 3; i++) {
- /*** start: check if player won ***/
- if (board[i][0] == player && board[i][1] == player && board[i][2] == player) return 1;
- if (board[0][i] == player && board[1][i] == player && board[2][i] == player) return 1;
- if (board[0][0] == player && board[1][1] == player && board[2][2] == player) return 1;
- if (board[0][2] == player && board[1][1] == player && board[2][0] == player) return 1;
- /*** end: check if player won ***/
- for (int j = 0; j < 3; j++)
- if (board[i][j] == 0) free++;
- }
- if (free == 0) return 0; //end of game - remis
- /*** start: check for loosing***/
- player = player == 1 ? 2 : 1; //switch player to opponent
- int best = -1;
- for (int i = 0; i < 3; i++) {
- for (int j = 0; j < 3; j++) {
- if (board[i][j] == 0) {
- board[i][j] = player;
- var score = move(board, player);
- board[i][j] = 0;
- if (score == 1) //opponent win - player loose!!!;
- return -1;
- best = score > best ? score : best;
- }
- }
- }
- return -1 * best; //bast can be -1 (not loose) , or 0 remis
- /*** end: check for loosing ***/
- }