מרכיבים של WCF
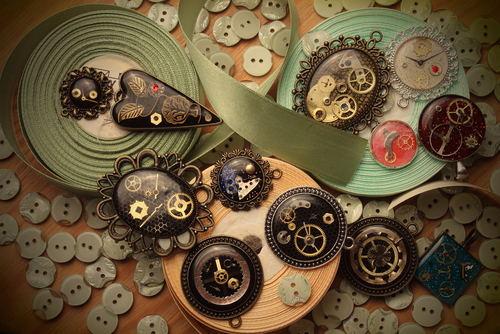
ממה מורכב WCF
Data contract and Service contract
- //
- //A data contract is a formal agreement between a service and a client that abstractly describes the data to be exchanged.
- //Examples from microsoft site:
- [ServiceContract]
- public interface ISampleInterface
- {
- // No data contract is required since both the parameter
- // and return types are primitive types.
- [OperationContract]
- double SquareRoot(int root);
- // No Data Contract required because both parameter and return
- // types are marked with the SerializableAttribute attribute.
- [OperationContract]
- System.Drawing.Bitmap GetPicture(System.Uri pictureUri);
- // The MyTypes.PurchaseOrder is a complex type, and thus
- // requires a data contract.
- [OperationContract]
- bool ApprovePurchaseOrder(MyTypes.PurchaseOrder po);
- }
- namespace MyTypes
- {
- [DataContract]
- public class PurchaseOrder
- {
- private int poId_value;
- // Apply the DataMemberAttribute to the property.
- [DataMember]
- public int PurchaseOrderId
- {
- get { return poId_value; }
- set { poId_value = value; }
- }
- }
- }
WCF Hosting:
- using (ServiceHost serviceHost = new ServiceHost(typeof (CalculatorService))) {
- serviceHost.Open();
- Console.WriteLine("The calculator service is ready.");
- Console.WriteLine("Press <ENTER> to terminate service.");
- Console.WriteLine();
- Console.ReadLine();
- }
Hosting configuration example:
- <?xml version="1.0" encoding="utf-8" ?>
- <configuration>
- <appSettings>
- </appSettings>
- <system.serviceModel>
- <services>
- <service name="TestService.CalculatorService"
- behaviorConfiguration="CalculatorServiceBehavior">
- <endpoint address="CalculatorService"
- binding="basicHttpBinding"
- contract="TestService.ICalculator" />
- <endpoint address="mex"
- binding="mexHttpBinding"
- contract="IMetadataExchange" />
- <host>
- <baseAddresses>
- <add baseAddress="http://localhost:8000/"/>
- </baseAddresses>
- </host>
- </service>
- </services>
- <behaviors>
- <serviceBehaviors>
- <behavior name="CalculatorServiceBehavior">
- <serviceMetadata httpGetEnabled="true"/>
- </behavior>
- </serviceBehaviors>
- </behaviors>
- </system.serviceModel>
- </configuration>
Channel factory client:
- var channelFactory = new ChannelFactory<IOrderServiceChannel>("httpEndPoint");
- var channel = channelFactory.CreateChannel();
- channel.PlaceOrder("Here are my order details...");
- channel.Close();
ClientBase (usable when: needed event-driven asynchronous model or remotely, when contracts not shared):
- using System;
- using System.ServiceModel;
- namespace MyMagazine.ServiceArticle
- {
- public class ProductClient
- : ClientBase<IProductBrowser>,
- IProductBrowser
- {
- #region IProductBrowser Members
- public ProductData GetProduct(
- Guid productID)
- {
- return Channel.GetProduct(productID);
- }
- public ProductData[] GetAllProducts()
- {
- return Channel.GetAllProducts();
- }
- public ProductData[] FindProducts(
- string productNameWildcard)
- {
- return Channel.FindProducts(
- productNameWildcard);
- }
- #endregion
- }
- }